Archived
This topic is now archived and is closed to further replies.
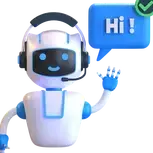
dbohdan's implementation
By
coders-irc_Bot, in Eggdrop
This topic is now archived and is closed to further replies.
By
coders-irc_Bot, in Eggdrop